Sean-Bradley Design-Patterns-In-Python: Common GOF Patterns implemented in Python
Table Of Content
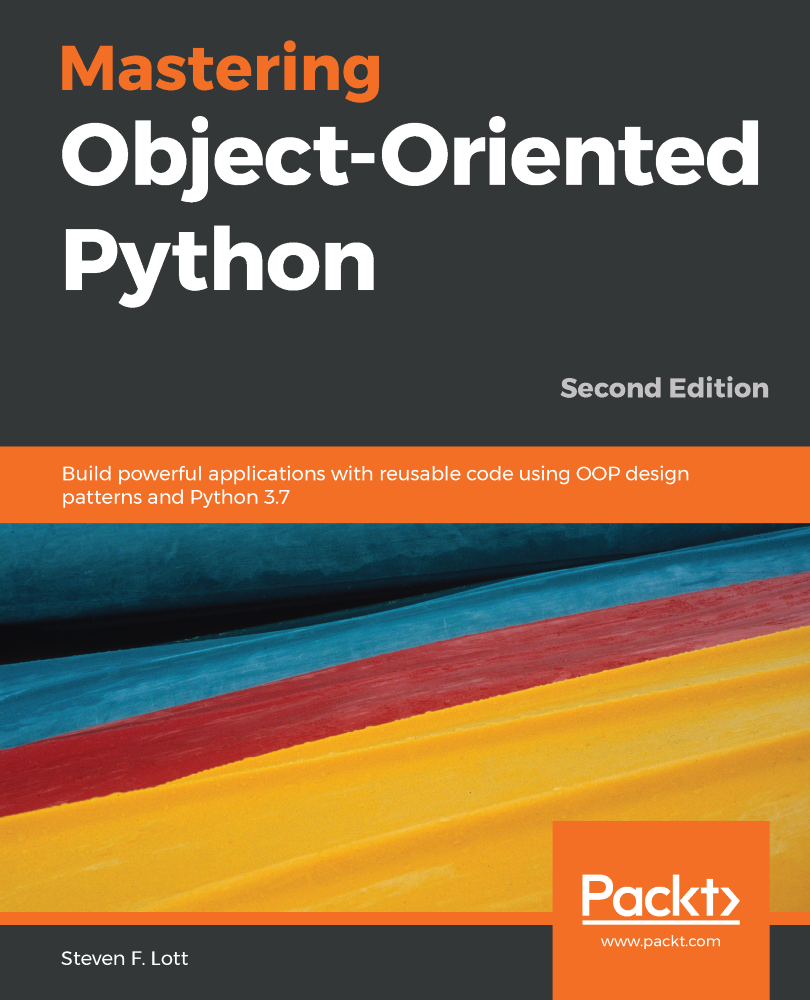
Someone wiser than I once said that Factory is built into Python. I have created fewer classes/subclasses compared to wrapping one class (or more often, several classes) in another class. These might not be Python patterns in the traditional sense, but these are rules that define the “Pythonic” approach to programming in the most elegant and useful fashion.
The Object-Oriented Programming (OOP) Paradigm
In Django, models define the data structure, views handle user interface logic, and controllers are automatically managed by the framework. In Flask, you can explicitly structure your codebase following the MVC pattern, enhancing maintainability and scalability. Say you're building a complex user interface (UI) for a software application. This UI has multiple components like buttons, text fields, and dropdown menus. For instance, selecting an option in a dropdown might enable or disable a button.
Step 3: Concrete Builder Classes
Meanwhile, your Square class breaks that assumption by changing the behavior promised by the object’s interface. That could have surprising and unwanted consequences, which would likely be hard to debug. In this snippet of code, you’ve defined Square as a subclass of Rectangle. As a user might expect, the class constructor takes only the side of the square as an argument. Internally, the .__init__() method initializes the parent’s attributes, .width and .height, with the side argument.
Software Design Pattern in other Programming Languages
He has full stack experience and has even developed a number of successful mobile apps and games. We've specified our type (MetaSingleton), the value to be assigned to the _instance field (cls), and other arguments we may be passing. If we try to do it directly we'll add a lot of dependencies branching in our code, and it may not even work at the end. The robot can be humanoid with four limbs and upward standing, or it can be animal-like with a tail, wings, etc. Now you have to make an entirely new class for the unemployed, which will take a completely different constructor! But now you don't know which constructor to call in a general case, much less which arguments to pass to it.
The Iterator pattern provides a solution by offering a way to access the elements of an aggregate object sequentially without exposing its underlying representation. In our code, this could look like a series of nested if-else statements, checking the type of issue and then directing it to the appropriate agent. But this approach quickly becomes unwieldy as more types of issues and specialists are added to the system. In this example, your FileManager class has two different responsibilities. It also deals with ZIP archives by providing the .compress() and .decompress() methods.
The .register_format(format, creator) method allows registering new formats by specifying a format value used to identify the format and a creator object. The creator object happens to be the class name of the concrete Serializer. This is possible because all the Serializer classes provide a default .__init__() to initialize the instances. The basic requirements for the example above are that you want to serialize Song objects into their string representation. It seems the application provides features related to music, so it is plausible that the application will need to serialize other type of objects like Playlist or Album.
Specializing Object Factory to Improve Code Readability
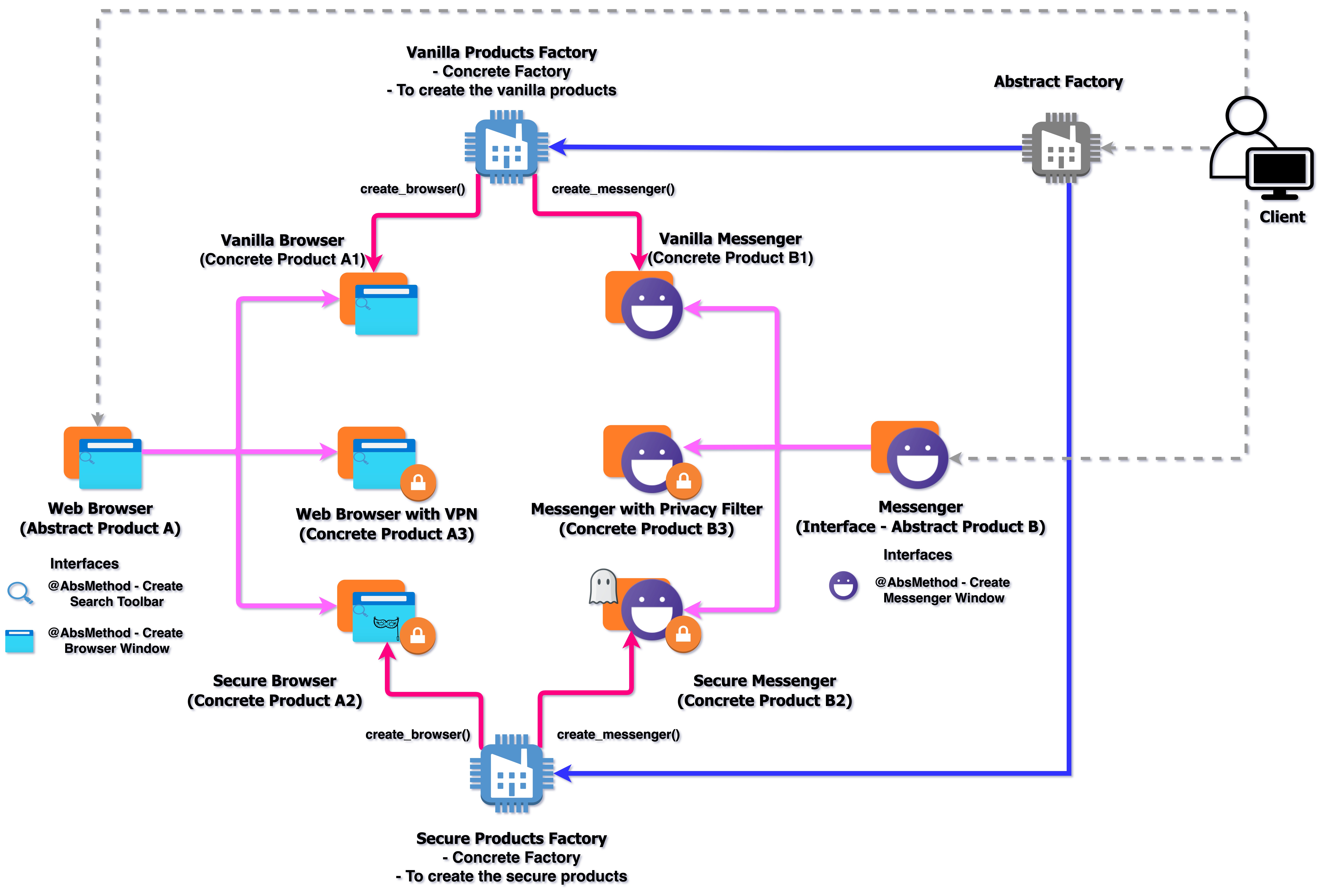
The creation of each concrete music service has its own set of requirements. This means a common initialization interface for each service implementation is not possible or recommended. You can see the basic interface of SerializerFactory in the implementation of ObjectSerializer.serialize(). The method uses factory.get_serializer(format) to retrieve the serializer from the object factory. As a matter of fact, the Song class doesn’t even know the goal is to convert the data to a string.
Languages
To get the most out of this tutorial, you must have a good understanding of Python object-oriented programming concepts, such as classes, interfaces, and inheritance. All of this means that our __call__() method will be called whenever a new object is created and it will provide a new instance if we haven't already initialized one. If we have, it will just return the already initialized instance. All of the usual classes you write, as well as the standard classes, have type as their object type. What this means is that type is a metaclass - other classes are instances of type, just like variable objects are instances of those classes. The main advantage of using this pattern is the control and predictability it offers.
We'll make a pool of objects that will be instantiated when we create the pool itself. Whenever we need to use the object of type MyClass, we'll acquire it from the pool, use it, and then release it back into the pool to be used again. MyClass is very useful and is often used throughout the project, but for short periods of time. Its instantiation and initialization are very expensive, however, and our program runs very slowly because it constantly needs to make new instances just to use them for a few operations. The purpose of using a metaclass in this case rather than a simpler implementation is essentially the ability to reuse the code.
Also, Spotify and Pandora require an authorization process before the service instance can be created. By implementing Factory Method using an Object Factory and providing a registration interface, you are able to support new formats without changing any of the existing application code. This minimizes the risk of breaking existing features or introducing subtle bugs. So far, we’ve seen the implementation of the client (ObjectSerializer) and the product (serializer).
More Pattern Matching in Python 3.10 by Alan Jones - Towards Data Science
More Pattern Matching in Python 3.10 by Alan Jones.
Posted: Mon, 14 Jun 2021 07:00:00 GMT [source]
These calls are commonly tied to expensive database operations, but can be other expensive processes. The Command pattern encapsulates a request as an object, thereby allowing you to parameterize clients with queues, requests, and operations. It promotes loose coupling between the sender and receiver of a request and enables the execution of operations without knowing the specifics of the requested action. The Command pattern is useful when you want to decouple objects that send requests from objects that process requests.
The reverse number pyramid pattern is a number pyramid reversed 180 degrees. But if you observe the code carefully then you will understand it easily. The left pascal triangle pattern is a little bit more complicated than the right pascal triangle pattern because you will have to deal with both spaces and stars. The left pascal triangle pattern is a mirror image of the right pascal triangle pattern.
An example would be changing the type of database you use (SQL or NoSQL) or changing the data structure you store your data in (dictionary or list). Clearly, super-class has properties attribute as dictionary data type, while sub-class is having the same attribute as list data type. But let’s say that there is a requirement to find all White coloured cars. Generally, the three groups above define how program elements relate to each other, how they are created, and how they communicate with each other. Python debugging refers to the process of resolving bugs (issues) that occur in Python programs. You can set breakpoints in your code and jump through them to view where your logic is flawed or your variables are set wrong.
It shows how patterns could be applied to real-world problems. We'll be creating a couple of NPC types for a fictional video game - a Shopkeeper, a Warrior and a Mage. In this guide, we'll take a look at the theory and implementation of the Prototype Design Pattern in Python and when you can benefit from leveraging it.
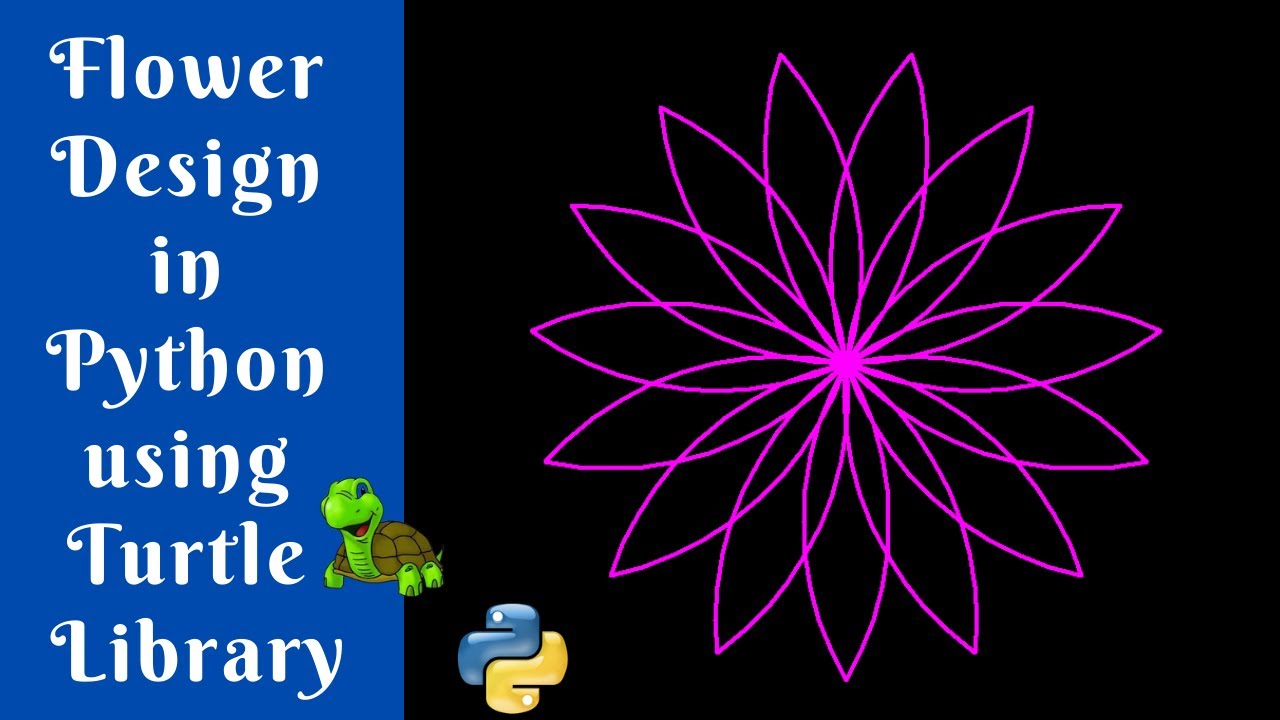
Finally, you define the API class to support retrieving the data from the REST API. Clearly, LandlinePhone inherited the properties that it doesn’t support and explicitly error has to be raised. Generally, If a base class have too many methods, possibly not all of the subclasses are going to need them. But due to inheritance, instances will be able to call these methods on all the subclasses. This means a lot of interfaces that are unused, unneeded and will result in bugs when they get accidentally called.
Comments
Post a Comment