Design Patterns in Python: Builder
Table Of Content

We'll be overriding the clone() method and actually provide an implementation for it. To copy the objects, we'll make use of the copy library, which is built-in into Python. The copy() method of the library performs a shallow copy of an object, while a deepcopy() creates a deep copy of an object. Depending on the structure of your objects - you'll prefer one or the other. Yes, Python supports design patterns just like any other programming language.
Behavioral Design Pattern:
It is important that the creator, in this case the Object Factory, returns fully initialized objects. Still, the current implementation is specifically targeted to the serialization problem above, and it is not reusable in other contexts. There is a wide range of problems that fit this description, so let’s take a look at some concrete examples. So, let’s check out how we decorate a method without using built-in Python functionality.
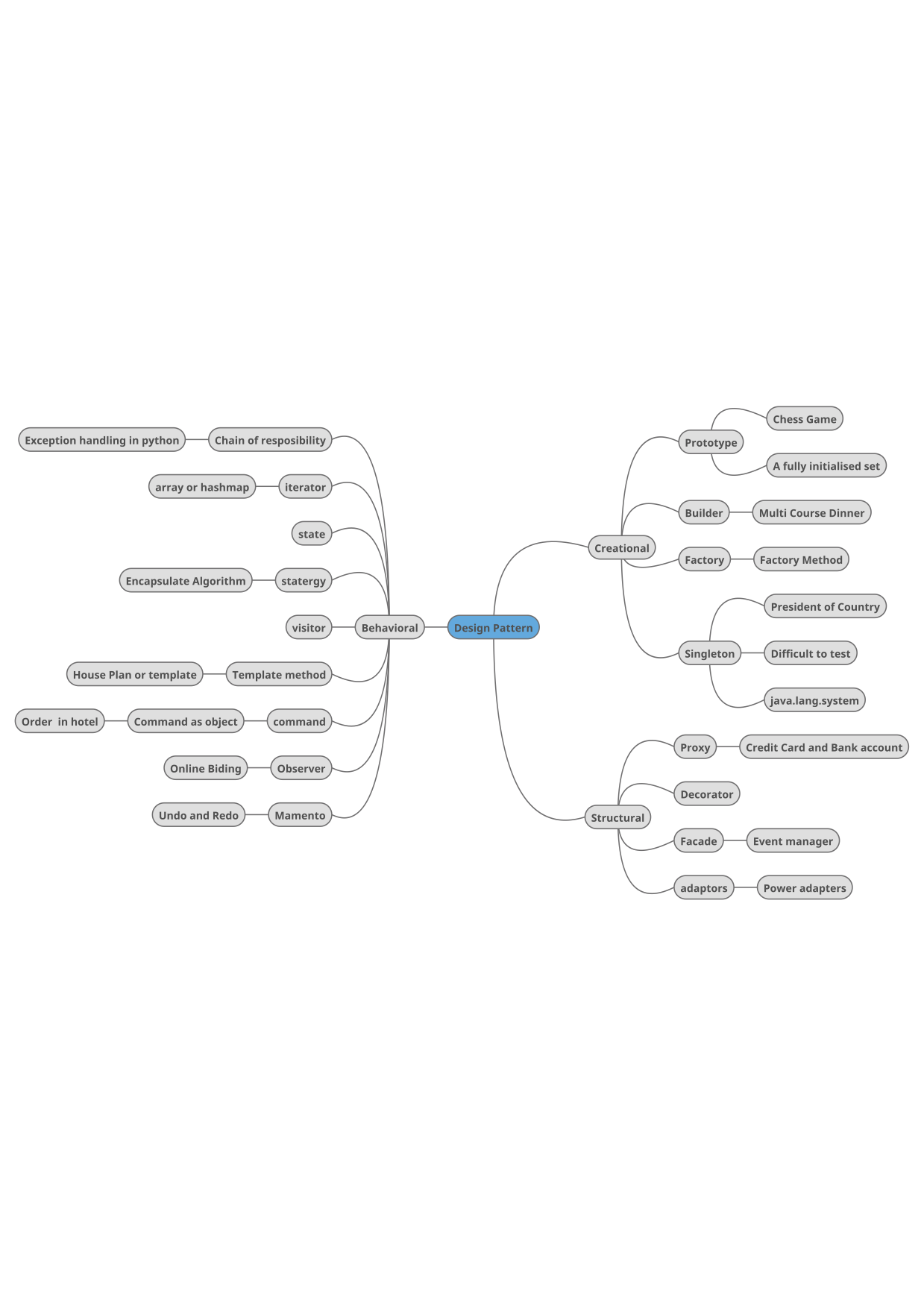
Technology Services
It also identifies the relationships which led to the simplification of the structure. In conclusion, design patterns form the backbone of effective Python software development. By aligning with object-oriented principles and core concepts, these patterns empower developers to build maintainable, flexible, and scalable applications.
BEFORE AND AFTER USING DESIGN PATTERNS -SIZE & CYCLOMATIC COMPLEXITY... - ResearchGate
BEFORE AND AFTER USING DESIGN PATTERNS -SIZE & CYCLOMATIC COMPLEXITY....
Posted: Wed, 01 Jul 2020 13:52:43 GMT [source]
Open-Closed Principle (OCP)
Creational Design Patterns, as the name implies, deal with the creation of classes or objects. Since both, super and sub-class, have different data types (implementations) we can’t apply any common procedure. That is where violating Liskov Substitution Principle starts causing trouble. The goal of the Open-Closed design principle is to minimize the changes in existing, tested code to prevent bugs and having to test everything all over again.
Up until now, we've covered Creational, Structural, and Behavioral design patterns. These foundational pillars have offered insights into crafting elegant, maintainable, and scalable Python applications. Yet, as we delve deeper into the nuances of Python, there emerge some design patterns that are unique to the language itself — the Python-specific design patterns. Facade is a popular structural design pattern that helps to simplify complex systems of classes, libraries, or frameworks. Facade acts as an interface between the client and the complex system enabling a simpler way of access.
To simulate this - we'll mock an expensive process call in the creation of our objects, lasting the entire three seconds. Then, using the Prototype Design Pattern - we'll create new objects while avoiding this limitation. While this approach is very intuitive and natural for us - things can get hectic real quick, just like the real world can. With many relationships, interactions and outcomes - it's hard to maintain everything in a coherent manner.
Creational Software Design Patterns in Python
Here, you first initialize FrontEnd using a Database object and then again using an API object. Every time you call .display_data(), the result will depend on the concrete data source that you use. Note that you can also change the data source dynamically by reassigning the .data_source attribute in your FrontEnd instance. Now you can add new shapes to your class design without the need to modify Shape.
Design Patterns in Python: Builder
There are of course a number of limitations, but that’s not the topic of this article. Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. Lets you fit more objects into the available amount of RAM by sharing common parts of state between multiple objects instead of keeping all of the data in each object. Provides a simplified interface to a library, a framework, or any other complex set of classes. Let's now create some pattern programs using alphabets instead of stars or numbers.
5 Free Advanced Python Programming Courses - KDnuggets
5 Free Advanced Python Programming Courses.
Posted: Wed, 24 Apr 2024 12:04:38 GMT [source]
If this principle is not followed, the result could be a long list of changes in depending classes and unnecessary hours of testing. Following are some of the commonly used design patterns in the software industry. The .create() method requires that additional arguments are specified as keyword arguments. This allows the Builder objects to specify the parameters they need and ignore the rest in no particular order. For example, you can see that create_local_music_service() specifies a local_music_location parameter and ignores the rest. Each service is initialized with a different set of parameters.
One of the most popular and widely accepted sets of standards for object-oriented design (OOD) is known as the SOLID principles. If we just get another factory as a parameter, we don't even need to know which class it produces. We just need to have a uniform factory method that returns a class guaranteed to have a certain set of behaviors.
Creational Design Patterns deal with creation of classes or objects. They're very useful for lowering levels of dependency and controlling how the user interacts with our classes. While design patterns are powerful tools, they should be used judiciously to avoid pitfalls.
After looking at 30 pattern program in python you have learned the basics of creating patterns. Now you can create your own patterns and make them look beautiful. Try the alphabet pattern program discussed in the next article. The complete code for the reverse alphabet pyramid pattern is given below.
You should also check out and master bridge and proxy design patterns, due to their similarity to adapter. Think how easy they are to implement in Python, and think about different ways you could use them in your project. You may also want to research Prototype, Builder and Factory design patterns. Again, we just demonstrated how implementing this wonderful design pattern in Python is just a matter of using the built-in functionalities of the language. The Singleton pattern is used when we want to guarantee that only one instance of a given class exists during runtime. Based on my experience, it’s easier to simply create one instance intentionally and then use it instead of implementing the Singleton pattern.
The number pyramid pattern is a pattern that is made of numbers and has a pyramid shape. The complete code for the right number triangle pattern is given below. The hollow triangle star pattern is a star pattern in the shape of the triangle made of stars but hollow. The right triangle star pattern is a star pattern in the shape of a triangle as shown above.
In this article, we will focus on building efficient and scalable applications in Python using popular design patterns. Design patterns are standards or conventions used to solve commonly occurring problems. Given Python’s highly flexible nature, design patterns are essential. The difference is in the interface that exposes to support creating any type of object.
Comments
Post a Comment